In this post, we will look at the various aspects of NestJS Swagger APIProperty.
This post directly builds up from the post about NestJS Swagger Configuration. So I will recommend you to go through that post and then continue with this one.
1 – The Need for NestJS Swagger APIProperty
The SwaggerModule automatically searches for all @Body(), @Query() and @Param() decorators in route handlers. Using these, it attempts to generate the API document. It also tries to create the model information by using reflection.
However, it is still not able to describe the various fields in the model successfully. See below screenshot:
As you can see, the Book schema in the Schemas section is empty even though we have fields in the Book class. To make the class properties visible in Swagger, we have to annotate them with @ApiProperty().
By the way, if you are interested in backend frameworks and concepts, you’d love the Progressive Coder newsletter where I explain such concepts in a fun and interesting manner.
Subscribe now and join along.
2 – The APIProperty Decorator
As discussed, we annotate the properties in our class with the @ApiProperty() decorator. See below:
import { ApiProperty } from "@nestjs/swagger";
export class Book {
@ApiProperty()
bookName: string;
@ApiProperty()
authorName: string;
@ApiProperty()
publishYear: number;
@ApiProperty()
isAvailable: boolean;
}
If we check the swagger definition now, we will see the below format:
Now, the schema definition has all the field names along with their data types.
3 – APIProperty Properties
Additionally, the @APIProperty() also allows us to set various other schema object properties. See below example where set the description of the property.
@ApiProperty({
description: 'The Name of the Book'
})
bookName: string;
If we wish to make a particular field as optional, we can also use the @ApiPropertyOptional() decorator.
@ApiProperty({
description: 'The Name of the Author'
})
@ApiPropertyOptional()
authorName: string;
We can also explicitly set the type of the property.
@ApiProperty({
description: 'The Year of Publishing',
type: Number
})
publishYear: number;
We can also default values for certain properties as below:
@ApiProperty({
description: 'Book Availability',
default: false
})
isAvailable: boolean;
4 – APIProperty Handling Enums
To describe a property that is of type, we have to set the enum property. In other words, we have to supply @ApiProperty() with an array of values.
See below example of another field we introduce.
@ApiProperty({
description: 'Type of Book',
enum: ['Fantasy', 'Science Fiction', 'Thriller']
})
genre: string;
If we check the swagger definition now with all the additions, we will the below schema.
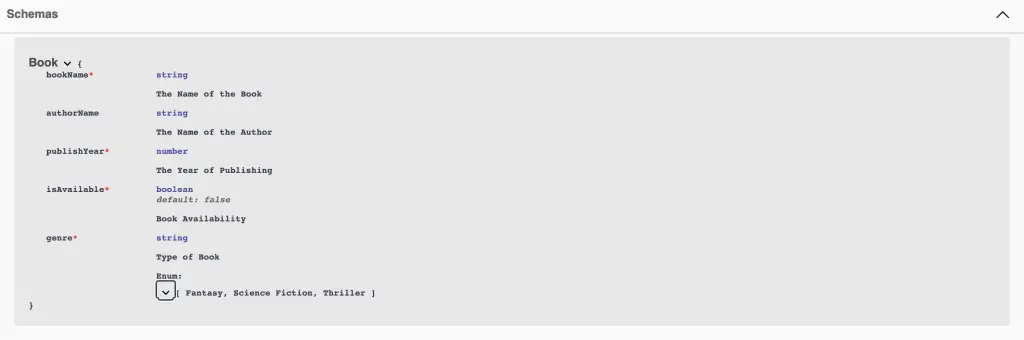
Below is the complete Book class for reference.
import { ApiProperty, ApiPropertyOptional } from "@nestjs/swagger";
export class Book {
@ApiProperty({
description: 'The Name of the Book'
})
bookName: string;
@ApiProperty({
description: 'The Name of the Author'
})
@ApiPropertyOptional()
authorName: string;
@ApiProperty({
description: 'The Year of Publishing',
type: Number
})
publishYear: number;
@ApiProperty({
description: 'Book Availability',
default: false
})
isAvailable: boolean;
@ApiProperty({
description: 'Type of Book',
enum: ['Fantasy', 'Science Fiction', 'Thriller']
})
genre: string;
}
5 – Conclusion
With this, we have successfully looked at NestJS Swagger APIProperty decorator and the various options it provides. These options help us customize the definitions in the swagger document to describe our APIs in a better way.
If you have any comments or queries, please mention in the comments section below.
Anyways, before we end this post, a quick reminder about the Progressive Code Newsletter where I explain backend frameworks and concepts in a fun & interesting manner so that you never forget what you’ve learned.
I’m 100% sure you’d love it.
Subscribe now and see you over there.
1 Comment
Moto · December 23, 2021 at 1:57 pm
Hi, thanks for the post.
Do you know if there is a way to use ApiProperty on parameter properties?
Would be nice
export class SomeDTO {
constructor(@ApiProperty() public name: string) {}
}