For any application, it is an important functionality to be able to provide its data to the consumer. REST APIs are one of the most popular ways to do so. In this post, we will look at the process of exposing repositories as REST resources using Spring Boot.
And by the way, Spring Boot makes it very easy to do so.
In the previous post, we inserted few records into our in-memory H2 database using Spring Boot. We used spring-boot-starter-data-jpa to do so. First, we created a Vehicle entity. After that, using the Command Line Runner, we inserted a couple of records into the corresponding table. Every time the application starts up, Spring will initialize a new instance of the database. Also, the two records will be inserted.
While this works perfectly fine, it is usually not the case in real apps. Normally, you would want your users to be able to insert records. For enabling them to do so, you need to provide an interface.
REST or Representational State Transfer is an architectural style that advocates the use of standard HTTP protocol to expose such an interface.
But how to create REST APIs?
Spring Data REST
In our starter application, we included a dependency that allows us to do so. See below:
1 2 3 4 | <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-rest</artifactId> </dependency> |
It is called spring-boot-starter-data-rest. This package makes it extremely easy to build hypermedia-based RESTful APIs. These APIs directly connect to the Spring Data repositories. Also, Spring automatically adds hypermedia capabilities to the exposed end-points.
Below is our entity class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | package com.progressivecoder.demo.springbootstarter.entities; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; import java.util.UUID; @Entity @Table(name = "vehicle") public class Vehicle { @Id private UUID id; private String vehicleIdentityNumber; private String make; private String model; public Vehicle() { } public UUID getId() { return id; } public void setId(UUID id) { this.id = id; } public String getVehicleIdentityNumber() { return vehicleIdentityNumber; } public void setVehicleIdentityNumber(String vehicleIdentityNumber) { this.vehicleIdentityNumber = vehicleIdentityNumber; } public String getMake() { return make; } public void setMake(String make) { this.make = make; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } @Override public String toString() { return "Vehicle{" + "id=" + id + ", vehicleIdentityNumber='" + vehicleIdentityNumber + '\'' + ", make='" + make + '\'' + ", model='" + model + '\'' + '}'; } } |
The Repository interface for Vehicle is as follows:
1 2 3 4 5 6 7 8 9 | package com.progressivecoder.demo.springbootstarter.repositories; import com.progressivecoder.demo.springbootstarter.entities.Vehicle; import org.springframework.data.repository.CrudRepository; import java.util.UUID; public interface VehicleRepository extends CrudRepository<Vehicle, UUID> { } |
Start the application now. Once it successfully starts, visit the URL http://localhost:8080/vehicles. You should see an output like below:
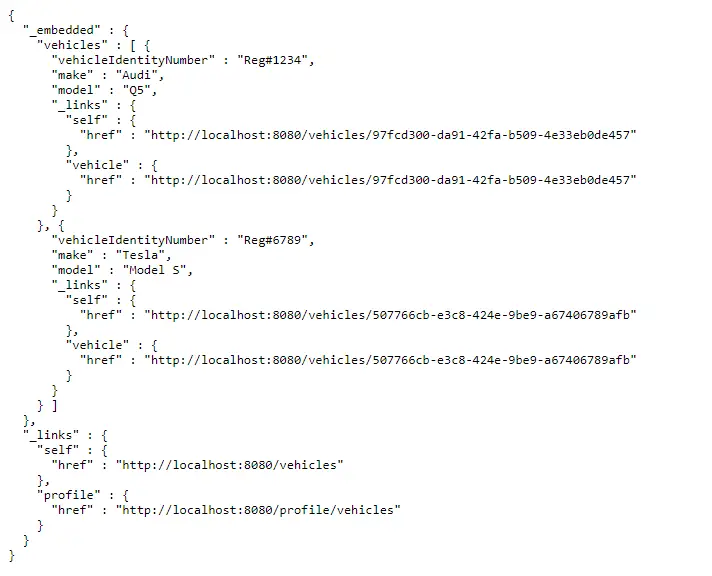
In the above screenshot, we can see a JSON payload. In the payload, we have the list of vehicles. Also, the _links section provides the links to the other URLs available for that resource. In this case, this means the URL to directly access an individual vehicle.
So just by adding the dependency in the POM.xml, you get all these capabilities for free. Isn’t that sweet?
Customizing the REST End-point
At this point, the REST endpoint exposed by our application is decided by Spring Boot. Since we defined our entity class as Vehicle, the end-point exposed is /vehicles.
However, many times we would like to customize this endpoint. To do so, the spring-boot-starter-data-rest provides an optional annotation @RepositoryRestResource.
This annotation can be used on the Repository interface definition. See below example:
1 2 3 | @RepositoryRestResource(collectionResourceRel = "cars", path = "cars") public interface VehicleRepository extends CrudRepository<Vehicle, UUID> { } |
Note the parameters collectionResourceRel and path. We have specified their value as cars. Due to this, the REST end-point exposed by our application will be /cars instead of /vehicles.
Run the application now and visit http://localhost:8080/cars.
You should see something like below:
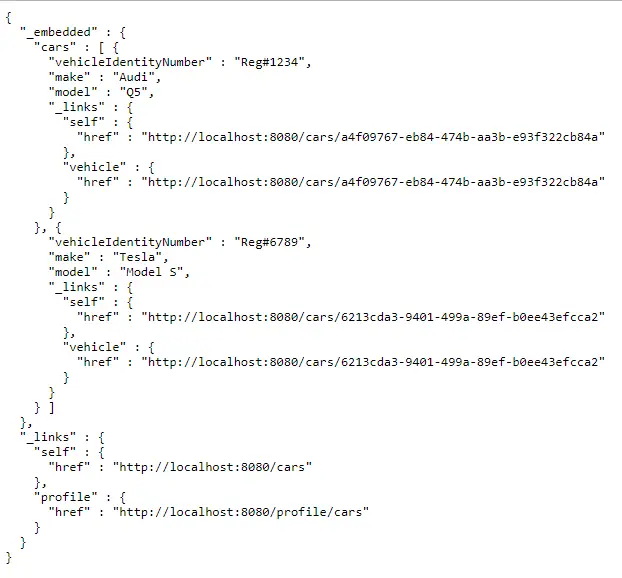
Selecting records based on condition
Many times, there is a requirement to select records based on some condition. In our example case, it could be based on the make of the vehicle. Or maybe the model.
Spring Data Rest makes it easy to do so.
To enable it, you need to add a method declaration to your repository interface as below:
1 2 3 4 5 | @RepositoryRestResource(collectionResourceRel = "cars", path = "cars") public interface VehicleRepository extends CrudRepository<Vehicle, UUID> { List<Vehicle> findByMake(@Param("make") String make); } |
In the above example, we are declaring a method that searches vehicles based on make. In other words, we are enabling our application to handle queries.
To see it in action, build the application now. Then, visit the URL http://localhost:8080/cars
This time your will see another end-point available in the hyperlink section.
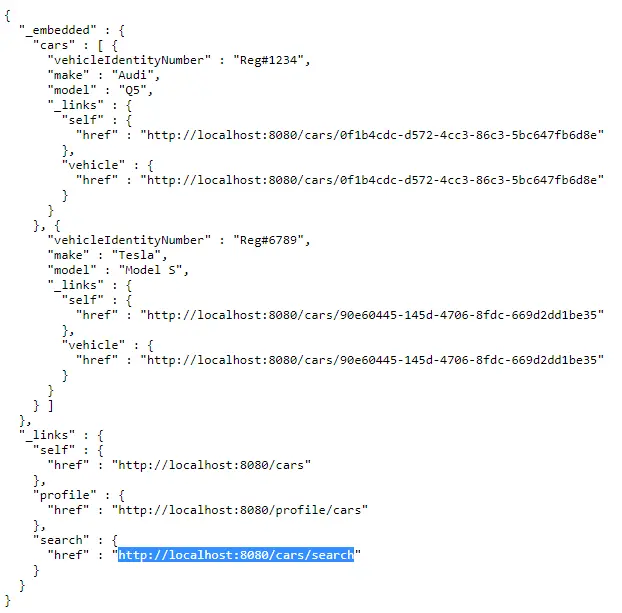
In order to actually query, you need to use the below endpoint:
http://localhost:8080/cars/search/findByMake?make=Tesla
This will give output as below:
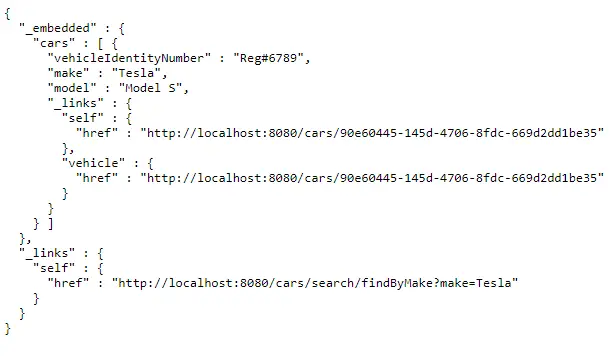
In the above screenshot, we can only see the vehicle with make of Tesla. This demonstrates that our query parameter is working properly.
As you can see, Spring Data REST is a powerful tool that allows us to rapidly expose REST interfaces to our repositories. The code for this can be found on Github.
In the next section, we will look at a more general approach of exposing REST APIs from our Spring Boot application.
2 Comments
Anonymous · September 18, 2020 at 9:14 am
is there any next section as i cannot see the hyperlink
Saurabh Dashora · September 18, 2020 at 9:19 am
Yes you can refer to the link. Also, updated the post with the correct link.