Axon 4.0 has made the shift from a being just a framework to a complete platform. The platform consists of the Axon Framework and the Axon Server. In this post, we will look at building microservices with Axon Server and Spring Boot.
Our focus will also be on setting up the Axon Server Dashboard and understand how it works and what type of useful information we can find on the dashboard.
Axon Architecture
Axon derives a lot of its principles from concepts of Domain Driven Design. Other concepts that inspire it are CQRS (Command Query Responsibility Segregation) and Event-Driven architecture.
We have already explored using Axon Framework in great detail in some of the below posts:
Event Sourcing
In Implementing Event Sourcing using Axon and Spring Boot – Part 1, we look at the general concept of event sourcing and a brief intro to Axon.
Inside the next post, Implementing Event Sourcing using Axon and Spring Boot – Part 2, we understand the step-by-step process of implementing event sourcing using Axon Framework and Spring Boot.
In Implementing Event Sourcing using Axon and Spring Boot – Part 3, we test our Event Sourcing application created in Part 2. We also see the event store in action.
Event Sourcing and CQRS
In Event Sourcing and CQRS with Axon and Spring Boot – Part 1, we understand how event sourcing and CQRS work in conjunction. Basically, we take a high-level view of these two concepts and plan the application we want to build.
Finally, in Event Sourcing and CQRS with Axon and Spring Boot – Part 2, we implement CQRS along with event sourcing using Axon Framework. Also, we explore Axon Framework 4.0 but without the Axon Server enabled. We also see the query store in action and how we can separate the commands and queries.
Axon Server
In the last implementation, we used Axon 4.0 but we excluded Axon Server from the dependencies. In this post, we will explore the server part of Axon and how it helps building and managing distributed applications.
A typical message-driven microservices application requires reliable communication between microservices. Also, this communication should be easy to monitor.
In Axon Framework, the internals of the communication are completely hidden from the developers. However, Axon Server provides a platform to the developers to handle this communication as well as monitor it.
In a typical setup, all applications register themselves to a central Axon Server. These applications will register their capabilities with the server. For example, one application can handle commands X and Y. Another application can handle some queries. Even multiple instances of the same application register with this central server.
Starting up the Axon Server
Axon Server can be started locally by downloading the zip file from the Axon site.
Then, we can execute the below command after entering the folder from the command prompt.
java -jar axonserver.jar
We should see something like below. The Axon Server will start at Port 8024.
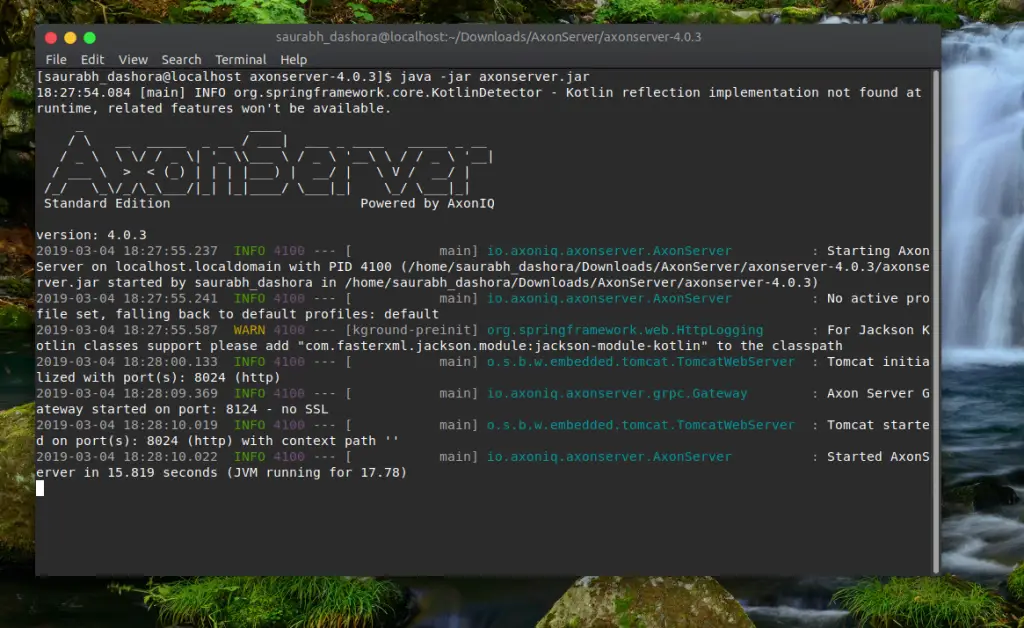
On visiting http://localhost:8024 now, we should see the Axon Server Dashboard.
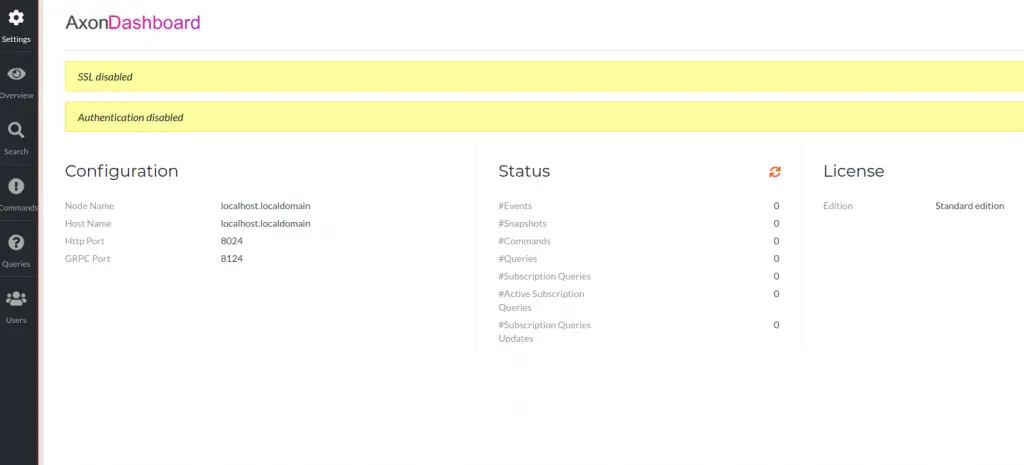
Connecting an Application to Axon Server
At this point, our Axon Server is running alone. There are no other applications for it to discover.
We will be using one of our applications built earlier to test with Axon Server. The code for this application is available on Github. If you want to have a detailed run down of how this application was built, refer to Event Sourcing and CQRS with Axon and Spring Boot – Part 1
The application uses Axon Version 4.0.3. Axon Server and Axon Framework are already part of this version and we don’t have to do much.
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.axonframework</groupId> <artifactId>axon-spring-boot-starter</artifactId> <version>4.0.3</version> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> <!-- Swagger --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
We can simply start this application using the command clean package spring-boot:run.
After the application has successfully started, we can visit the Axon Dashboard. On clicking the Overview tab on the left side, we can see that our application has been discovered by the Axon Server. See below screenshot.
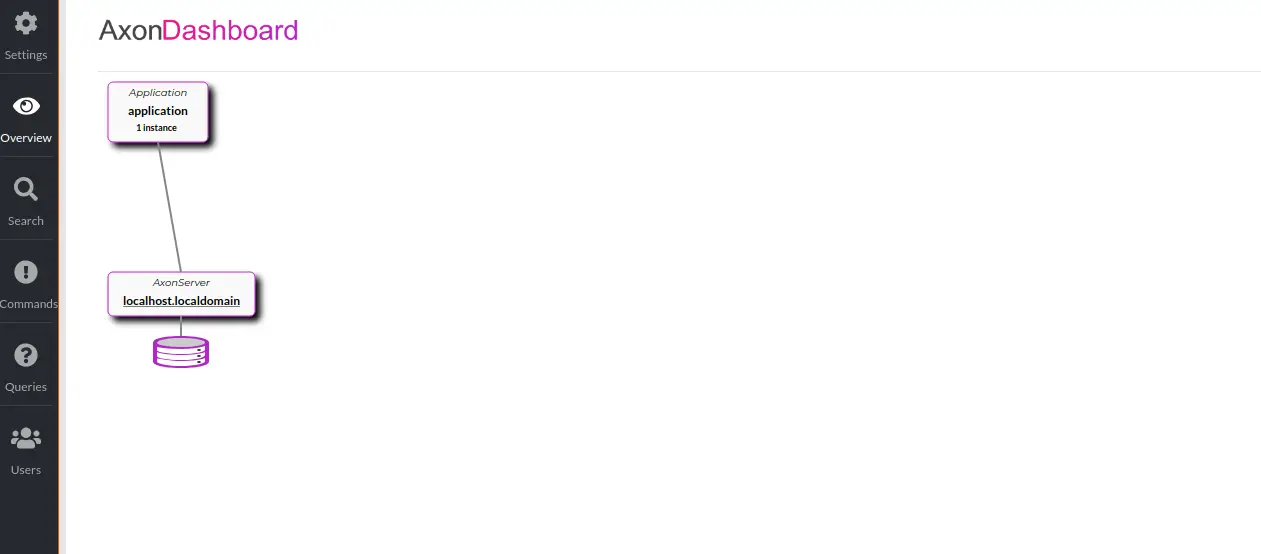
Testing the Application with Axon Server
Now that the Axon Server is up and running, we can test our application and see what the dashboard displays.
The application we have can be easily tested using Swagger-UI. If you want a detailed run down of the testing process using Swagger-UI, refer to Implementing Event Sourcing with Axon and Spring Boot – Part 3
Basically, we just fire a few a command supported by our application such as Create an Account.
If we see the Commands tab in the server dashboard, we can now see that one command was registered.
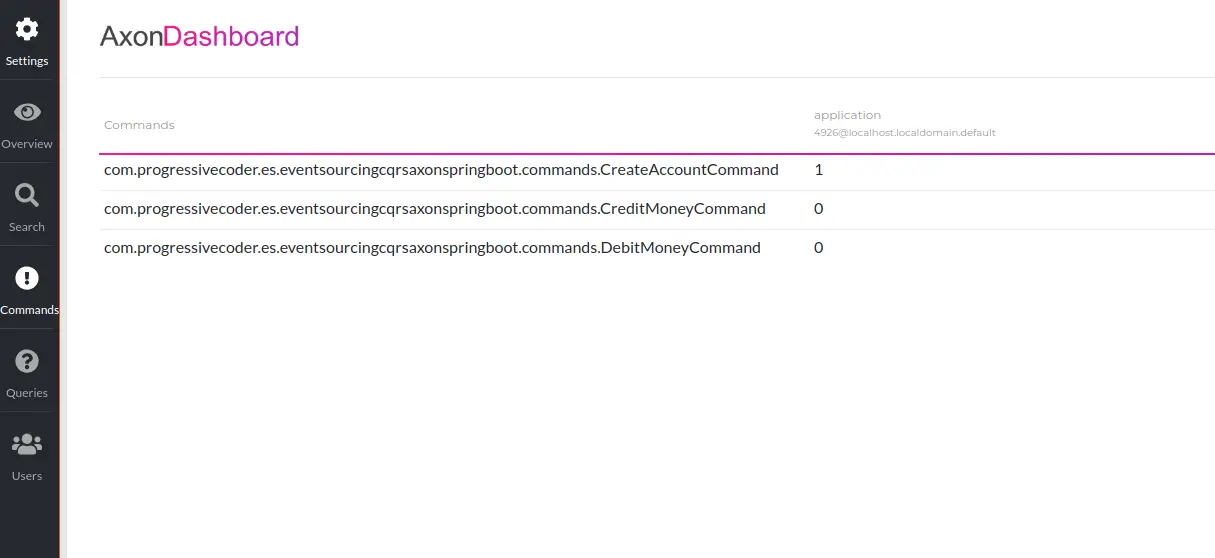
The overview page also shows the total count of commands and also the number of events that were triggered. We can see 2 events and 1 command.
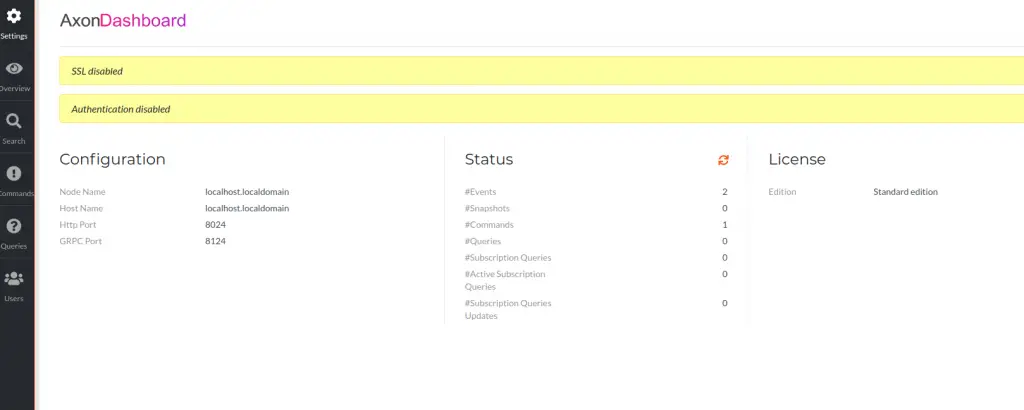
The server dashboard also provides a way to inspect the event store. Basically, we can access the event store by clicking Search tab on the left hand side of the page. On pressing Search with empty search string, we can see all the events logged in the application.
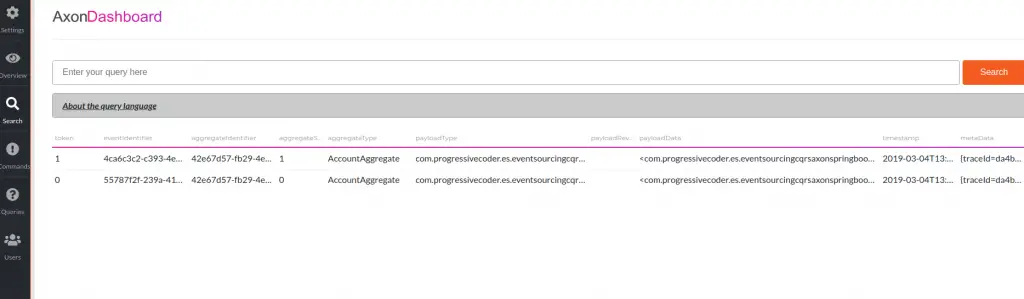
We can also write queries by using the specific query language specified in the documentation below the search bar.
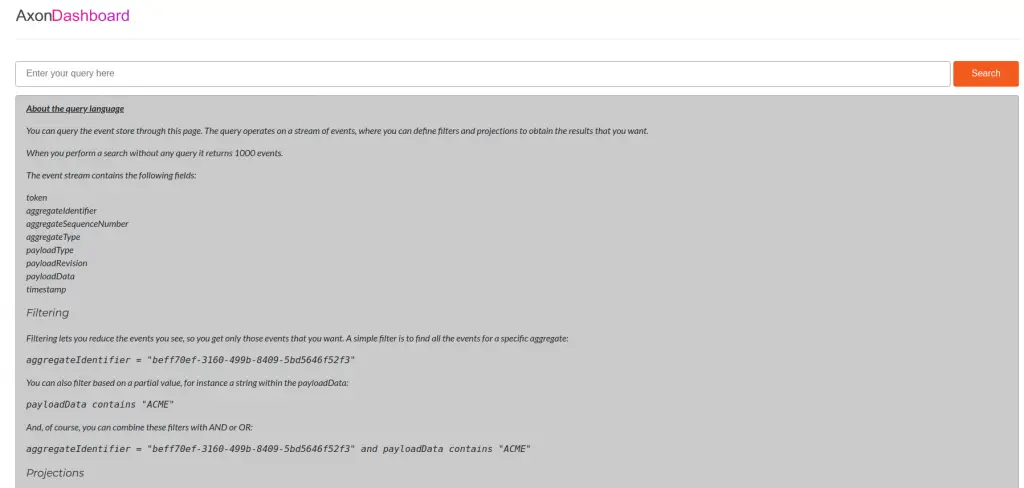
Conclusion
In this post, we successfully connected our Spring Boot application built using Axon Framework with the Axon Server.
The code for the application that we used is available on Github.
Axon makes it easy to build distributed applications by doing the heavy-lifting in terms of handling the message streams. It also provides a dashboard view allowing developers to monitor all the applications connected to the Axon Server.
6 Comments
Ravi · August 14, 2019 at 12:12 pm
How to use Rabbit-Mq in place of Axon Server to Send the Messages
Saurabh Dashora · August 15, 2019 at 3:25 am
Hi Ravi,
You can exclude the Axon server dependency from the POM and instead wire up RabbitMQ. To publish messages to RabbitMQ, you could you use something like Spring Cloud Stream to make things easy.
Luis · April 23, 2020 at 2:06 am
In regards to the previous example “Event Sourcing and CQRS with Axon and Spring Boot”, I did that locally without any problem, but after removing the exclusion for axon-server-connector in that project in order to connect this out to Axon Server as it is proposed in this article, even though I can see the application in axon server’s dashboard (overview section). When I ran a command that triggers an event, this is not shown on the board as well as when I ran a query. By taking a look at the database (h2) I realized that the DOMAIN_EVENT_ENTRY table does not exist. Is that correct? It does not, why the table is not created? Because when the axon connector is commented, it worked previously. To be honest I was not able to realize why.
Maybe you can give me your point of view.
Thanks in advance,
Luis.
Saurabh Dashora · April 28, 2020 at 3:10 am
Hi Luis,
When you use axon-server-connector in your project (i.e. you don’t exclude it explicitly), then the events are not logged in the H2 database of the application. The events are available in the Axon Server itself.
You can refer to the below post to see how that works:
https://progressivecoder.com/building-microservices-with-axon-server-and-spring-boot
Deep Shah · February 4, 2022 at 1:06 pm
Hi thanks for the tutorial !!!.
I have doubt like ….
I want to delete all the events in Axon store …
Is there any way to delete them..
As In my Project I have to do that
Saurabh Dashora · February 5, 2022 at 1:25 am
Hi Deep, Not sure about that. You may have to check Axon documentation!