Application security is one of the most important aspects of building production-level Spring Boot Microservices. An insecure application is vulnerable to malicious access. Spring Boot Security Starter module helps us implement basic security mechanism in our application.
Let’s see it in action.
The Dependencies
Spring Boot has a starter module that enables security in our applications.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
Just by including this dependency in our POM.xml file, the Spring Boot Security module is activated. When Spring Boot application starts up and it finds this module in the classpath, it will bootstrap the necessary security related functionality.
To see it in action, we can start-up our application using the below command:
clean package spring-boot:run
After the application starts up and we visit the URL http://localhost:8080/swagger-ui.html, we will be presented with a login screen.
You can login to this screen using default username as user. The default password is randomly generated by Spring. It will be available in the start-up logs.

Below is how the login screen might look like depending on the version of Spring Boot.
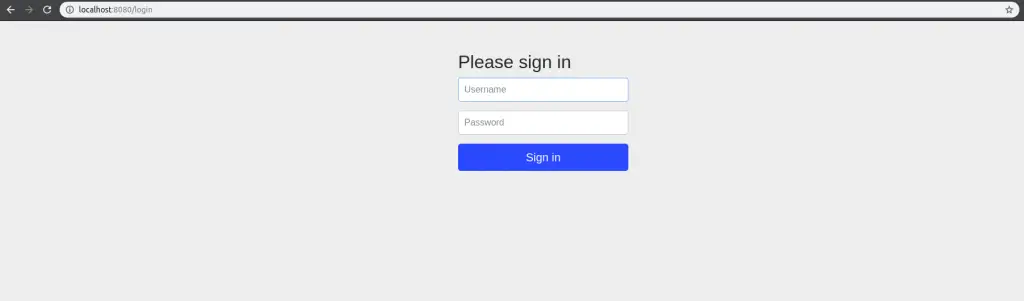
Customizing the Spring Boot Security
While the above approach is also securing the application in principle, it is not a good idea to simply use the default username and password. Usually username and passwords are set by the application developer. They should not change each time the application starts up.
We can handle the above situation pretty easily by defining our own username and password values in the application.properties file.
#Security
spring.security.user.name=application-user
spring.security.user.password=testpassword
Now, when you start up the application using the earlier command, Spring Boot Security will automatically wire up the username and password set in this file as the application username and password.
Securing the UserName and Password
However, even this approach is not enough for a real application. Some of the issues with this approach are as follows:
- The username and password is basically available in plain-text. If you commit the source code to a repository, anyone who has access to the repository can see the username and password. In other words, this is a critical security issue.
- Another problem is that in this approach username and password are always the same. Often, an application will move through several environment such as Development, User Testing and Production. Usually, we would want to assign different username and password for different environments.
To fix the above issues, we need to have a different strategy for managing the username and password. Basically, the username and password should be tied to the environment. Also, these should be bound to the application only at run-time.
To do so, we will parameterize the username and password fields. See below changes to the application.properties file.
#Security
spring.security.user.name=${username}
spring.security.user.password=${password}
Basically, we are telling Spring Boot to expect values for user.name and user.password properties to come from the variable username and password.
Then, while starting up the application, we can set these values as environment variables by using the below command:
clean package spring-boot:run -Dusername=application-user -Dpassword=testpassword
As evident, we are setting username and password variables to their respective values.
Even if you are running the application as a JAR file, you can pass the environment variables as below:
java -jar spring-boot-starter-0.0.1-SNAPSHOT.jar -Dusername=application-user -Dpassword=password
The above approach basically helps managing user credentials for Spring Boot Security in a better way. Also, parameterizing helps in tweaking these properties during run-time.
Conclusion
Spring Boot Security module is the simplest way to enable basic security mechanism for our Spring Boot Microservices. In this post, we have done exactly that. You can refer to the code for this post on Github.
However, the approach described here is still naive from an overall security point of view. In this approach, we are just authenticating users based on a hard-coded username and password. This is far from optimum.
Ideally, security should be based on a user and their respective role so that you can have more fine-grained control over the resources exposed from your application. We need a more robust solution.
Fortunately, Spring Boot provides us with tools to achieve the same.
One of them is Spring Boot Security using UserDetailsService. Basically, in this post, we look at how to integrate our security solution with a user database.
The next one is Spring Boot Method Level Security. Basically, here we look at how to add authorization to our Spring Boot application.
Happy Learning!
1 Comment
Binh Thanh Nguyen · February 23, 2020 at 5:02 pm
Thanks, nice tips